Download FPDF from here:-
https://www.fpdf.org
Keep the fpdf.php file in your working directory, keep the font directory in the same place.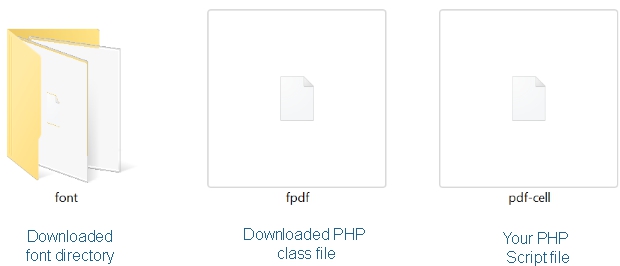
Run this code , you are ready with your first pdf file created from FPDF class.
<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->Cell(80,10,'Hello World!');
$pdf->Output('my_file.pdf','I'); // Send to browser and display
?>
Above code will display the string, we can manage this output by adding border , alignment, colour , link etc..<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->Cell(80,10,'Hello World!',1,0,'R',false,'https://www.google.com');
$pdf->Output('my_file.pdf','I'); // send to browser and display
?>
In the above code we have used cell to add our text to the pdf file with border, alignment , background fill and a link. Here is the details of Cell

Try to change the script by adding backgroud color like this.
$pdf->SetFillColor(1,255,255);
$pdf->Cell(80,10,'Hello World!',1,0,R,true,'https://www.google.com');
$pdf->Output('my_file.pdf','D');
Note : Cell function background fill option must be set to trueAdding font colour by SetTextColor()
<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->SetFillColor(1,99,255); // input R ,G , B
$pdf->SetTextColor(255,254,254);// input R , G , B
$pdf->Cell(80,10,'Hello World!',1,0,C,true,'https://www.plus2net.com');
$pdf->Output('my_file.pdf','I'); // Send to browser and display
?>
With border colour by SetDrawColor() and border size by SetLineWidth()
<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->SetFillColor(1,99,255); // input R ,G , B
$pdf->SetTextColor(255,254,254);// input R , G , B
$pdf->SetDrawColor(255,1,1);// input R , G , B
$pdf->SetLineWidth(1);
$pdf->Cell(80,10,'Hello World!',1,0,C,true,'https://www.plus2net.com');
$pdf->Output('my_file.pdf','I'); // Send to browser and display
?>
We can add border in any side or in all sides or we can remove the borders of the cell by assigning different values.
Example : To add border to Left , Right and top we can give the option as LRT, similarly we can assign border to bottom only by giving the option as B.Saving the PDF file
$pdf->Output('my_file','D');
to get output directly. This line will force download to user machine as pdf file my_file.pdf. We can change this output to save the file in local ( server ) side machine.$pdf->Output('my_file.pdf','I'); // Send to browser and display
$pdf->Output('my_file.pdf','D'); // Force file download with name given ( name can be changed )
$pdf->Output('my_file.pdf','F'); // Saved in local computer with the file name given
$pdf->Output('my_file.pdf','S'); // Return the document as a string.
MultiCell:
MultiCell to display text with line breaks:
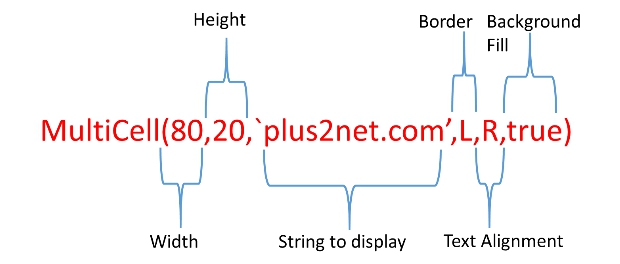
Text string wrap into next line after reaching the border of the MultiCell. In case of Cell the text crosses and flows out of the Cell border. MultiCell is used when we are not sure about the length of the string to display.
<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->SetX(50); // abscissa or Horizontal position
$pdf->Cell(60,10,'This is Cell - Welcome to plus2net.com',1,1,L,false);
$pdf->Ln(40); // Line gap
$pdf->SetX(50); // abscissa of Horizontal position
$pdf->MultiCell(60,10,'This is MultiCell - Welcome to plus2net.com',LRTB,L,false);
$pdf->Output('my_file.pdf','I'); // send to browser and display
?>
MultiCell Border:
1 : All borders
0 : No Border
L : Only Left boarder
RT: Only Right and Top Border
BTL: Only Bottom Top and Left side
You can continue with other combinations of the borders.
MultiCell Text Alignment:
C: center
R: right
J: justification (default value is J)
<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->SetX(50); // abscissa of Horizontal position
$pdf->MultiCell(100,10,'Alignment = L',1,L,false);
$pdf->Ln(20); // Line gap
$pdf->SetX(50); // abscissa of Horizontal position
$pdf->MultiCell(100,10,'Alignment = R',1,R,false);
$pdf->Ln(20);
$pdf->SetX(50);
$pdf->MultiCell(100,10,'Alignment = C',1,C,false);
$pdf->Ln(20);
$pdf->SetX(50);
$pdf->MultiCell(100,10,'Demo About MultiCell Alignment = J',1,J,false);
$pdf->Output('my_file.pdf','I'); // send to browser and display
?>
MultiCell and fill:
<?Php
require('fpdf.php');
$pdf = new FPDF();
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$pdf->SetX(50);
$pdf->MultiCell(100,10,'SetFillColor is not set, value =false',1,J,false);
$pdf->SetFillColor(1,255,255);
$pdf->Ln(20);
$pdf->SetX(50); // abscissa of Horizontal position
$pdf->MultiCell(100,10,'$pdf->SetFillColor(1,255,255);',1,C,true);
$pdf->SetFillColor(255,255,1);
$pdf->Ln(20); // Line gap
$pdf->SetX(50); // abscissa of Horizontal position
$pdf->MultiCell(100,10,'$pdf->SetFillColor(255,255,1);',1,C,true);
$pdf->SetFillColor(255,1,255);
$pdf->Ln(20);
$pdf->SetX(50);
$pdf->MultiCell(100,10,'$pdf->SetFillColor(255,1,255);',1,C,true);
$pdf->Output('my_file.pdf','I'); // send to browser and display
?>
Position of X and Y in MulitCell:

We can control the starting point of MultiCell by using top margin and left margin. In the example below we have displayed the X and Y position by reading the value using GetX and GetY functions.
We have used PHP Math function round() to get rounded value of X and Y coordinates.
<?Php require('fpdf.php');
$pdf = new FPDF();
$pdf->SetLeftMargin(50); // before adding a page
$pdf->SetTopMargin(30); // before adding a page
$pdf->AddPage();
$pdf->SetFont('Arial','B',16);
$h=15; // default height of each MultiCell
$w=100;// Width of each MultiCell
$y=$pdf->GetY(); // Getting Y or vertical position
$x=$pdf->GetX(); // Getting X or horizontal position
$pdf->MultiCell($w,$h,'X='.round($x).',Y='.round($y),LRTB,L,false);
$y=$pdf->GetY();
$x=$pdf->GetX();
$pdf->MultiCell($w,$h,'X='.round($x).',Y='.round($y),LRTB,L,false);
$y=$pdf->GetY();
$x=$pdf->GetX();
$pdf->MultiCell($w,$h,'X='.round($x).',Y='.round($y),LRTB,L,false);
$y=$pdf->GetY();
$x=$pdf->GetX();
$pdf->MultiCell($w,$h,'X='.round($x).',Y='.round($y).' ( Added more text inside MultiCell for text to Wrap around )',LRTB,L,false);
$y=$pdf->GetY();
$x=$pdf->GetX();
$pdf->MultiCell($w,$h,'X='.round($x).',Y='.round($y),LRTB,L,false);
$pdf->Output('my_file.pdf','I'); // send to browser and display
?>
No comments:
Post a Comment